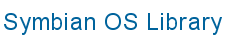
![]() |
![]() |
|
CMdaAudioPlayerUtility
provides a simple interface
to open, play, and obtain information from sampled audio data. The audio data
can be supplied either in a file, a descriptor or a URL. One significant
difference between this class and CMdaAudioRecorderUtility
(that also provides play methods) is that multiple audio files can be played
within a single instance with this class.
For the purposes of this description, the playing process has been broken down into the following sections.
Construction and Opening -
describes the options available for initially constructing the
CMdaAudioPlayerUtility
object, then using subsequent open commands
to play other audio files.
Configuration - describes the various configuration settings such as volume and balance settings that can be queried or set.
Plug-in Control - describes the information and commands that can be sent to or retrieved from plug-in controllers.
Meta data control - describes the methods available for meta data manipulation.
Playing - describes the methods available for playing audio clips.
When using CMdaAudioPlayerUtility
you can specify
the name of the file, descriptor or URL to play in a variety of ways. As with
CMdaAudioRecorderUtulity
and
CMdaAudioConverterUtulity
this class can automatically detect the
file format and codec of most audio clips with the exception of raw audio. To
play raw audio, it is necessary to first create the player object using
NewL()
and then use OpenUrlL()
or
OpenAndPlayUrlL()
.
The following construction and open statements are provided:
Construction statements for initialising the object with and without a specified audio file.
static CMdaAudioPlayerUtility* NewL(MMdaAudioPlayerCallback& aCallback, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality);
static CMdaAudioPlayerUtility* NewFilePlayerL(const TDesC& aFileName, MMdaAudioPlayerCallback& aCallback, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality, CMdaServer* aServer=NULL);
static CMdaAudioPlayerUtility* NewDesPlayerL(const TDesC8& aData, MMdaAudioPlayerCallback& aCallback, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality, CMdaServer* aServer=NULL);
static CMdaAudioPlayerUtility* NewDesPlayerReadOnlyL(const TDesC8& aData, MMdaAudioPlayerCallback& aCallback, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality, CMdaServer* aServer=NULL);
Use NewL()
if you just want to instantiate the
player object without having to specify an audio clip.
Use NewFilePlayerL()
,
NewDesPlayerL()
or
NewDesPlayerReadOnlyL()
if you want to instantiate the
player object and pre-load an audio clip ready for playing.
As soon as initialisation of the audio player utility is complete,
successfully or otherwise, the callback function
MMdaAudioPlayerCallback::MapcInitComplete()
is called.
Note: Additional audio clips can be played using the same instance of the player utility by using any of the open statements described below.
Open statements for opening audio clips after the player object has been created.
OpenFileL(const TDesC& aFileName);
OpenDesL(const TDesC8& aDescriptor);
OpenUrlL(const TDesC& aUrl, TInt aIapId = KUseDefaultIap, const TDesC8& aMimeType=KNullDesC8);
If an audio clip is already open and playing, use
Stop()
followed by Close()
to unload
the audio clip before specifying new audio data to open.
Open statements for opening and automatically playing audio clips after the player object has been created.
OpenAndPlayFileL(const TDesC& aFileName, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality);
OpenAndPlayDesL(const TDesC8& aDescriptor, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality);
OpenAndPlayUrlL(const TDesC& aUrl, TInt aIapId = KUseDefaultIap, const TDesC8& aMimeType=KNullDesC8, TInt aPriority=EMdaPriorityNormal, TMdaPriorityPreference aPref=EMdaPriorityPreferenceTimeAndQuality);
If an audio clip is already open and playing, use
Stop()
followed by Close()
to unload
the audio clip before specifying new audio data to open.
Following the constructing and opening of the audio clip, configuration adjustments can be made using the methods described below.
The configuration related methods are:
Methods for controlling the volume settings of the audio device are
GetVolume()
, SetVolume()
,
MaxVolume()
and SetVolumeRamp()
.
Methods for controlling the balance of audio channels are
GetBalance()
and SetBalance()
.
SetPriority()
, sets the priority of the audio
device. This priority setting is used to arbitrate between multiple objects
trying to access a controller.
You can govern the relationship with a video plug-n in various ways:
ControllerImplementationInformationL()
-
allows applications to query implementation information about the controller
plugin in use.
CustomCommandSync()
- allows applications to
send custom commands to controller plugins.
RegisterForAudioLoadingNotification()
-
registers to receive notifications of audio clip loading/rebuffering.
GetAudioLoadingProgressL()
- gets the progress
of audio clip loading/rebuffering.
Some audio formats enable the use of meta data, enabling the player of an audio clip to retrieve information that is held within the clip itself. This meta data is usually used to store information such as copyright information, creator, creation date and so on.
CMdaAudioPlayerUtility
provides two meta data
methods that enable you to:
Get the number of meta data entries in a clip with
GetNumberOfMetaDataEntries()
.
Retrieve a specific meta data entry within a clip with
GetMetaDataEntryL()
.
Note: it is not possible to set meta data using this class. If you need
to set meta data, use CMdaAudioConvertUtility
instead.
This class is intended for playing audio data only. Whereas
CMdaAudioRecorderUtility
does contain some player methods,
this class is far better suited to playing audio clips. This is because
CMdaAudioPlayerUtility
does not contain the overheads
required to support recording and because it is capable of playing more than
one clip within the same instance.
The play related methods are:
Play()
- start playback of audio data from the
current position. When playing of the audio sample is complete, successfully or
otherwise, the callback function
MMdaAudioPlayerCallback::MapcPlayComplete()
is called.
SetRepeats()
- set the number of times to
repeat playing back the same piece of audio data.
Duration()
- determine the duration of the
audio clip in microseconds.
SetPlayWindow()
and
ClearPlayWindow()
- set and clear the start and end
position within the audio clip from where to start and stop playing audio data.
GetPosition()
and
SetPosition()
retrieves or sets the current playing
position within the audio clip.
Pause()
enables you to pause playback; the
position within the audio clip is maintained in case a subsequent
PlayL()
is issued.
Stop()
stops playback of the audio sample as
soon as is possible and calls the callback function
MMdaAudioPlayerCallback::MapcPlayComplete()
. If playback
is already complete, this function has no effect.
Close()
closes all related controllers.
Multi Media Framework Client Overview - general overview of the various components of the MMF Client API and the functionality they provide.
Audio Recording, Conversion and Playing - advanced audio file manipulation features; specifically, the ability to record, convert and playback sound clips as well as to manipulate meta data.
Audio Recording - introduction to the audio recording class.
Audio Conversion - introduction to the audio conversion class.
Audio Streaming - the interface to streaming sampled audio.
Audio Tone Player - a simple interface for tone generation (synthesized sounds) that is supported on all audio-capable devices.
Video Recording and Playing - video manipulation features; specifically, the ability to record and playback video clips as well as to manipulate meta data.
Video Recording - introduction to the video recording class.
Video Playing - introduction to the video playing class.