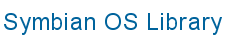
![]() |
![]() |
|
Dial a call with CTelephony::DialNewCall()
,
passing it the number to dial in a CTelephony::TTelNumber
.
The method starts the dialling process.
CTelephony::DialNewCall()
returns a
CTelephony::TCallId
that identifies the call. This will be
either CTelephony::EISVCall1
or
CTelephony::EISVCall2
. You need this ID to perform
operations such as putting the call on hold and hanging up. It tells
CTelephony
's methods which call to operate on.
To control whether you want the call's recipient to know your
identity, pass CTelephony::DialNewCall()
a
CTelephony::TCallParamsV1
. You have three options: hide
your identity, show your identity, or use the phone's default setting. To read
your phone's default setting, see Caller ID - Withholding your number.
CTelephony::DialNewCall()
is asynchronous; use
CTelephony::EDialNewCallCancel
to cancel it.
This example dials the number 123456789
and allows the
remote party to see the phone's number:
#include <e32base.h>
#include <Etel3rdParty.h>
_LIT(KTheNumber, "123456789");
class CClientApp : public CActive
{
private:
CTelephony* iTelephony;
CTelephony::TCallId iCallId;
public:
CClientApp(CTelephony* aTelephony);
void SomeFunction();
private:
/*
These are the pure virtual methods from CActive that
MUST be implemented by all active objects
*/
void RunL();
void DoCancel();
};
CClientApp::CClientApp(CTelephony* aTelephony)
: CActive(EPriorityStandard),
iTelephony(aTelephony)
{
//default constructor
}
void CClientApp::SomeFunction()
{
CTelephony::TTelNumber telNumber(KTheNumber);
CTelephony::TCallParamsV1 callParams;
callParams.iIdRestrict = CTelephony::ESendMyId;
CTelephony::TCallParamsV1Pckg callParamsPckg(callParams);
iTelephony->DialNewCall(iStatus, callParamsPckg, telNumber, iCallId);
SetActive();
}
void CClientApp::RunL()
{
if(iStatus==KErrNone)
{} // The call has been dialled successfully;
// iCallId contains the call's ID, needed when controlling the call.
}
void CClientApp::DoCancel()
{
iTelephony->CancelAsync(CTelephony::EDialNewCallCancel);
}
Remember to link to the Etel3rdParty.lib
and
euser.lib
libraries.
You can check the voice line's status by calling
CTelephony::GetLineStatus()
. The call has not connected
until the status is CTelephony::EStatusConnected
.
This describes how to dial a second call while another is in progress.
Put the first call on hold
This is described in Hold a call.
If the first call was not dialled or answered by you then you
cannot control it, and so you cannot put it on hold. If it is not on hold
already then you will have to wait until the it is put on hold (i.e. the
Voice line status
changes to CTelephony::EStatusHold
).
Dial the second call
This is the same as dialling a call in
Dial a call.
CTelephony::AnswerIncomingCall()
will return a different call ID
to the first call.
Remember that dialling a call is an asynchronous operation: you do not know whether you have successfully connected until the asynchronous operation has completed. If the operation fails, remember to resume the first call; see Resume a call.
As a result, the first call is on hold, and the phone user is talking to the second caller.