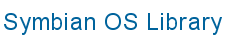
![]() |
![]() |
|
Location:
BITDEV.H
Link against: bitgdi.lib
class CFbsScreenDevice : public CFbsDevice;
A graphics device interface that provides direct access to the screen, without the mediation of the window server.
The interface adds sprite support to the CFbsDevice
base class.
MGraphicsDeviceMap
- Interface class for mapping between twips and device-specific units (pixels)
CBase
- Base class for all classes to be instantiated on the heap
CGraphicsDevice
- Specifies the interface for concrete device classes
CBitmapDevice
- Defines an abstract interface for the capabilities and attributes of a bitmapped graphics device
CFbsDevice
- Abstract base class for graphics devices to which bitmaps and fonts can be drawn
CFbsScreenDevice
- A graphics device interface that provides direct access to the screen, without the mediation of the window server
Defined in CFbsScreenDevice
:
CancelSprite()
, ChangeScreenDevice()
, DrawSpriteBegin()
, DrawSpriteEnd()
, GetPalette()
, GetPixel()
, GetScanLine()
, HardwareBitmap()
, HideSprite()
, HideSprite()
, HorizontalPixelsToTwips()
, HorizontalTwipsToPixels()
, NewL()
, NewL()
, NewL()
, PaletteAttributes()
, ScreenNo()
, SetAutoUpdate()
, SetPalette()
, ShowSprite()
, ShowSprite()
, SizeInTwips()
, Update()
, Update()
, VerticalPixelsToTwips()
, VerticalTwipsToPixels()
, ~CFbsScreenDevice()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CBitmapDevice
:
CreateBitmapContext()
Inherited from CFbsDevice
:
AddFile()
,
CreateContext()
,
DisplayMode()
,
DisplayMode16M()
,
DrawingBegin()
,
DrawingEnd()
,
FontHeightInPixels()
,
FontHeightInTwips()
,
GetDrawRect()
,
GetFontById()
,
GetNearestFontInPixels()
,
GetNearestFontInTwips()
,
GetNearestFontToDesignHeightInPixels()
,
GetNearestFontToDesignHeightInTwips()
,
GetNearestFontToMaxHeightInPixels()
,
GetNearestFontToMaxHeightInTwips()
,
GraphicsAccelerator()
,
NumTypefaces()
,
Orientation()
,
RectCompare()
,
ReleaseFont()
,
RemoveFile()
,
SetBits()
,
SetCustomPalette()
,
SetScalingFactor()
,
SizeInPixels()
,
TypefaceSupport()
,
iBitBltMaskedBuffer
,
iDrawDevice
,
iFbs
,
iGraphicsAccelerator
,
iOrientation
,
iScreenDevice
,
iSpare
,
iTypefaceStore
Inherited from MGraphicsDeviceMap
:
PixelsToTwips()
,
TwipsToPixels()
static IMPORT_C CFbsScreenDevice *NewL(const TDesC &aLibname, TDisplayMode aDispMode);
Creates a new CFbsScreenDevice object.
|
|
static IMPORT_C CFbsScreenDevice *NewL(const TDesC &aLibname, TDisplayMode aDispMode, TRgb aWhite);
Creates a new CFbsScreenDevice object.
|
|
static IMPORT_C CFbsScreenDevice *NewL(TInt aScreenNo, TDisplayMode aDispMode);
Creates a new CFbsScreenDevice object.
|
|
virtual IMPORT_C ~CFbsScreenDevice();
Frees all resources owned by the object prior to its destruction.
virtual IMPORT_C void GetScanLine(TDes8 &aBuf, const TPoint &aStartPixel, TInt aLength, TDisplayMode aDispMode) const;
Copies a scanline into a buffer.
This implements the pure virtual function CBitmapDevice::GetScanLine()
.
|
virtual IMPORT_C void GetPixel(TRgb &aColor, const TPoint &aPixel) const;
Gets the RGB colour of an individual pixel on a bitmapped graphics device.
This implements the pure virtual function CBitmapDevice::GetPixel()
.
|
virtual IMPORT_C TInt HorizontalPixelsToTwips(TInt aPixels) const;
Converts a horizontal dimension from pixels to twips.
This implements the pure virtual function MGraphicsDeviceMap::HorizontalPixelsToTwips()
.
|
|
virtual IMPORT_C TInt VerticalPixelsToTwips(TInt aPixels) const;
Converts a vertical dimension from pixels to twips.
This implements the pure virtual function MGraphicsDeviceMap::VerticalPixelsToTwips()
.
|
|
virtual IMPORT_C TInt HorizontalTwipsToPixels(TInt aTwips) const;
Converts a horizontal dimension from twips to pixels.
This implements the pure virtual function MGraphicsDeviceMap::HorizontalTwipsToPixels()
.
|
|
virtual IMPORT_C TInt VerticalTwipsToPixels(TInt aTwips) const;
Converts a vertical dimension from twips to pixels.
This implements the pure virtual function MGraphicsDeviceMap::VerticalTwipsToPixels()
.
|
|
IMPORT_C void SetAutoUpdate(TBool aValue);
Sets or unsets auto-update for the screen.
|
virtual IMPORT_C TSpriteBase *HideSprite() const;
Hides the sprite over the whole screen.
|
virtual IMPORT_C TSpriteBase *HideSprite(const TRect &aRect, const TRegion *aClippingRegion) const;
Hides the sprite within a particular region.
|
|
virtual IMPORT_C void ShowSprite(TSpriteBase *aSprite) const;
Shows the specified sprite over the whole screen.
|
virtual IMPORT_C void ShowSprite(TSpriteBase *aSprite, const TRect &aRect, const TRegion *aClippingRegion) const;
Shows the specified sprite within a particular region.
|
IMPORT_C void ChangeScreenDevice(CFbsScreenDevice *aOldDevice);
Changes the screen device.
|
virtual IMPORT_C void PaletteAttributes(TBool &aModifiable, TInt &aNumEntries) const;
Gets the palette attributes of the device.
|
virtual IMPORT_C void SetPalette(CPalette *aPalette);
Sets the device palette to the specified palette.
|
virtual IMPORT_C TInt GetPalette(CPalette *&aPalette) const;
Gets the devices current palette.
|
|
IMPORT_C void Update(const TRegion &aRegion);
Forces any out of date region of the screen to update, and additionally forces the specified region to update.
|
virtual IMPORT_C TSize SizeInTwips() const;
Gets the size of the device area, in twips.
This implements the pure virtual function CGraphicsDevice::SizeInTwips()
.
|
IMPORT_C RHardwareBitmap HardwareBitmap();
Creates and returns a hardware bitmap (a bitmap which can be drawn to by a graphics accelerator whose operations may be implemented in hardware or software), whose handle is to the screen.
This allows the caller to draw to the screen like any other hardware bitmap.
This function may not be supported on all hardware. If unsupported, it returns an RHardwareBitmap
with a handle of zero.
The hardware bitmap can be used to draw directly to the screen. Use it to create a TAcceleratedBitmapSpec
object, which can either be used to get a TAcceleratedBitmapInfo
, or can be passed to a graphics operation (an instance of a class derived from class TGraphicsOperation
) e.g. a bitblt to copy one part of the screen to another.
Direct screen access must only be carried out in combination with the Window Server's direct screen access classes; i.e. only use the hardware bitmap on the CFbsScreenDevice which you get from CDirectScreenAccess, and not from your own CFbsScreenDevice.
|
IMPORT_C TInt ScreenNo() const;
Query the screen number to which the object refers.
|